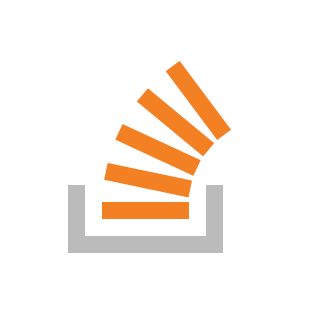
Streamline Compilation: How to Master Global Flags in Visual Studio
Managing compilation flags across multiple projects in Visual Studio can quickly become a headache. Tired of endless configuration combinations? You're not alone! Let's explore intelligent strategies to get those flags under control and boost your development workflow.
The Challenge: Compilation Chaos
Visual Studio's built-in Configuration Manager is a starting point.
- It lets you define flags and switch configurations.
- Combining multiple flags leads to a configuration explosion.
- Managing 10+ flags becomes a configuration nightmare.
Solution 1: The Central Header File Approach
Mimic the C++ #include
strategy for centralized flag management.
Create a Dedicated Header File
- Create a
CompilationFlags.h
file (or similar). - Define your global flags within this file using
#define
. - Include this header in all relevant project files.
// CompilationFlags.h
#ifndef COMPILATION_FLAGS_H
#define COMPILATION_FLAGS_H
#define ENABLE_FEATURE_A
// #define ENABLE_FEATURE_B // Comment out to disable
#define DEBUG_MODE
#endif
Benefits: Single source of truth, easy toggling, and improved maintainability.
Solution 2: Inter-Project Constant Propagation
Explore ways to share constants between projects during builds.
Research Project Dependencies and Build Order
- Ensure the project defining the constants builds first.
- Investigate if you can expose the constants as public symbols.
- Reference the output of the defining project in dependent projects.
Caveat: This might involve more complex project setup, but could be viable for certain scenarios.
Solution 3: Leverage MSBuild Properties and Conditions
Harness the power of MSBuild for advanced flag control.
Define Properties in a Common Props File
- Create a
.props
file (e.g.,GlobalFlags.props
). - Define MSBuild properties with conditional values.
- Import this
.props
file into all relevant project files.
Use Properties in Conditional Compilation
- Use the MSBuild properties within your code using preprocessor directives.
#ifdef $(EnableFeatureA)
// Code for Feature A
#endif
Benefits: Configuration-aware flags, centralized control through MSBuild, and flexible conditional compilation.
Solution 4: Environment Variables
Define the compilation flags via system environment variables.
How To Set Up the Environment Variables
- Define a set of variables associated with a certain feature.
- Each feature can be turned on by setting the environment variable to
true
, and can be turned off if set tofalse
.
#ifdef _WIN32
#define GET_ENV(var) getenv(var)
#else
#define GET_ENV(var) std::getenv(var)
#endif
#ifdef GET_ENV("ENABLE_FEATURE_A")
#define ENABLE_FEATURE_A
#endif
Benefits: Simple to turn on and off features without recompilation.
Best Practices for Flag Management
No matter which approach you choose, keep these tips in mind:
- Naming Conventions: Use clear and consistent names for your flags (e.g.,
ENABLE_FEATURE_X
,DEBUG_MODE
). - Documentation: Document what each flag controls and its intended use.
- Version Control: Track changes to your flag definitions using your version control system.
- Testing: Thoroughly test your code with different flag combinations.
Take Control of Your Compilation
Don't let compilation flags bog you down. By implementing a strategic approach, you can maintain a clean, organized, and efficient development environment. Happy coding!