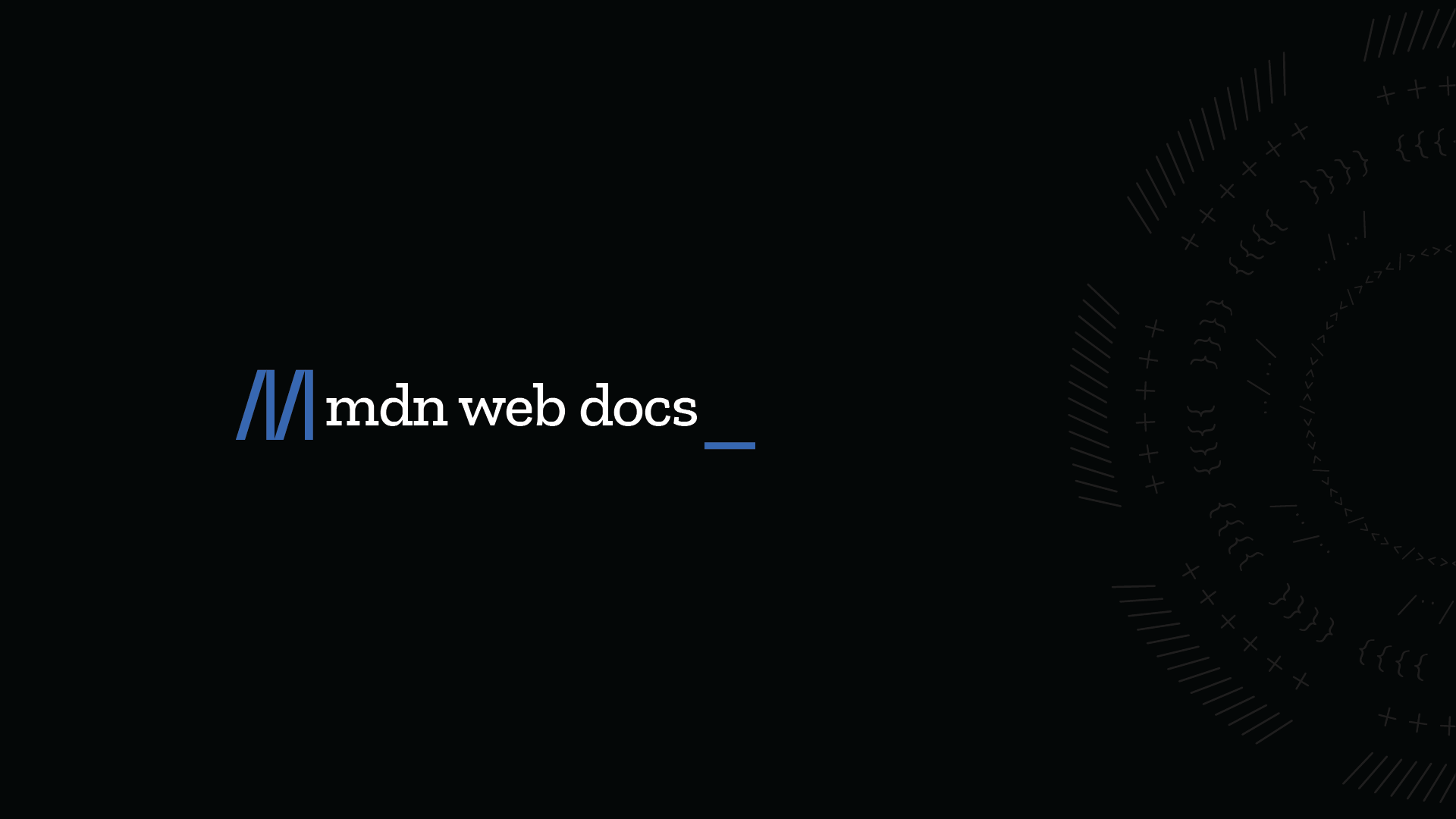
Master the Sensor APIs: A Developer's Guide to Accelerometer, Gyroscope, and More
Unlock the power of device sensors in your web applications! This comprehensive guide explores the Sensor APIs, providing you with the knowledge and code snippets to integrate motion, orientation, and environmental data seamlessly. Learn about the Accelerometer, Gyroscope, Magnetometer, and other sensor interfaces with practical examples.
What are the Sensor APIs?
The Sensor APIs offer a standardized way to access device sensors within web applications.
- These APIs provide interfaces for accessing data from various sensors.
- They help create immersive and responsive user experiences by sensing the environment and device motion.
- You can create innovative features that react to the user's physical movements or ambient conditions.
Understanding Sensor API Concepts
The Sensor APIs expose device sensors consistently to the web platform through a set of interfaces built to a common design
- The
Sensor
interface is the base for all other sensor types but isn't used directly. - Subclasses like
Accelerometer
andGyroscope
provide specific sensor data. - Some sensors are low-level (directly representing hardware), while others are high-level (fusing data from multiple sensors).
Feature Detection: Is the Sensor Available?
Before diving into sensor usage, ensure the API and underlying sensor are available. Here's how:
- API Detection: Check if the sensor interface exists in the
window
object. - Defensive Programming: Instantiate the sensor in a
try...catch
block to handle potential errors. - Always confirm the existence of the actual sensor, don't rely on API presence alone.
Defensive Programming: Handling Sensor Errors
Robust error handling is crucial when working with sensors. Implement these strategies:
- Instantiation Errors: Use
try...catch
to catch errors during sensor creation. - Runtime Errors: Listen for the
error
event on the sensor object. - Graceful Degradation: Provide a fallback experience if the sensor is unavailable or permission is denied.
Permissions and Permissions Policy: Requesting Access
User permission is essential to access sensor data. Respect user privacy by following these steps:
- Permissions API: Use
navigator.permissions.query()
to check sensor permissions. - Error Handling: Listen for
SecurityError
events if permission is denied. - Permissions Policy: Ensure your server's Permissions Policy allows sensor access.
Reading Sensor Data: Getting Real-Time Updates
Access sensor readings through the reading
event. Control the reading frequency for optimal performance:
- Reading Event: Listen for the
reading
event on the sensor object. - Frequency Control: Set the
frequency
option in the sensor constructor (readings per second). - Hardware Limits: Real reading frequency depends on device capabilities.
Sensor APIs: Available Interfaces
Explore the various sensor interfaces available:
AbsoluteOrientationSensor
: Device orientation relative to Earth's coordinate system.Accelerometer
: Acceleration along three axes.AmbientLightSensor
: Ambient light level.GravitySensor
: Gravity applied to the device.Gyroscope
: Angular velocity along three axes.LinearAccelerationSensor
: Acceleration without gravity.Magnetometer
: Magnetic field detection.RelativeOrientationSensor
: Device orientation without Earth's reference.Sensor
: Base class for all sensors.SensorErrorEvent
: Provides error information.
Browser Compatibility
Always check browser compatibility before implementing Sensor APIs. Refer to MDN's browser compatibility tables for each sensor interface to ensure cross-device functionality.